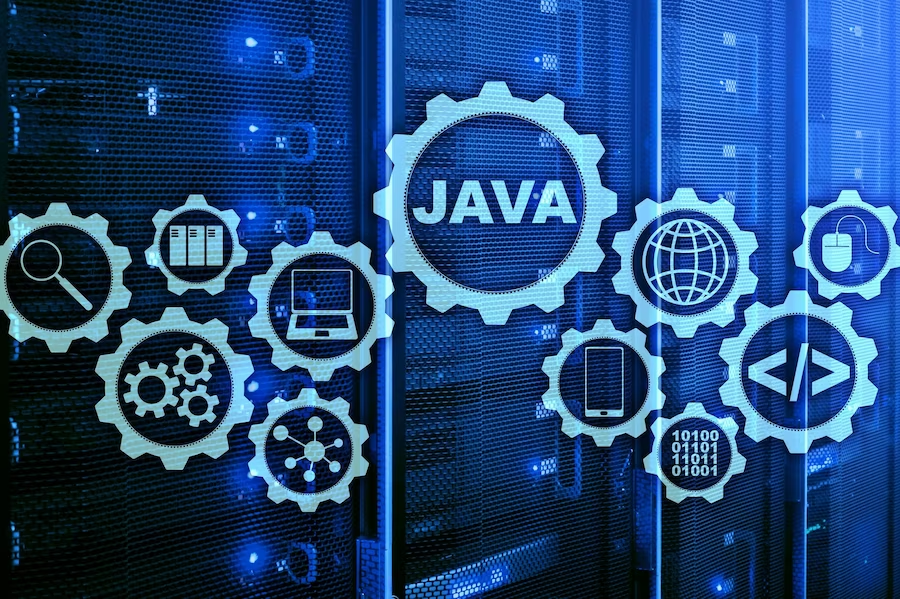
Conquer Your Next Interview: Top 5 Java Programs for Freshers
Apr 16, 2024 5 Min Read 4631 Views
(Last Updated)
We all know about the deadly cycle of needing the experience to get a job and needing a job to get some experience right? Like how do I show you the experience if none of you give me the chance to prove myself? *rolls eyes*
That is why Technical Rounds have always been my personal favorite, I get to portray my programming skills and the language or tech knowledge I possess without any barriers.
So if you’re a fresher looking for a job in the Java programming field, it is important to thoroughly prepare yourself for the interview process.
One of the key aspects of this preparation is knowing the top Java interview programs that employers often ask candidates to solve.
In this article, I will introduce you to some of the most commonly asked programming questions in the field for freshers, and provide tips on how to solve them, so do follow along throughout!
Table of contents
- Why you should read this article...
- Key Concepts to Understand Before the Interview:
- Object-Oriented Programming (OOP) Interview Questions in Java
- Java Advanced Interview Questions
- Top 5 Must-Know Java Interview Programs for Freshers
- Fibonacci Series
- Check if a Number is Prime
- String Palindrome
- Bubble Sort
- Armstrong Number
- Tips for Solving Interview Questions Efficiently
- Additional Resources for Interview Preparation
- Common Mistakes to Avoid During Interviews
- FAQs
- What are the basic programs asked in interviews for freshers in Java?
- How to prepare for Java interview for freshers?
- How to practice Java for interview?
Why you should read this article…
Before we dive into the top Java interview programs, let’s first understand why it is important to know them.
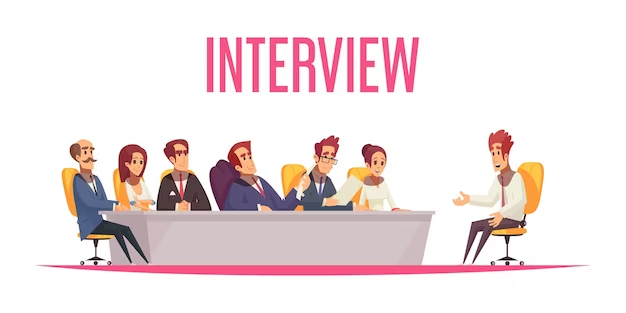
As a fresher, you may not have much practical experience in the field (aka the irritating cycle of repetition), but you can still demonstrate your knowledge of the language and its concepts through your answers to interview questions.
By showing that you are familiar with commonly used Java programs that form the basis of the language, you can prove to the interviewer that you have a strong foundation in the language and are capable of efficiently solving problems using Java.
Accelerate your Java programming career with GUVI’s Java Course. Upskill your programming language and learn everything related to Java.
Key Concepts to Understand Before the Interview:
Object-Oriented Programming (OOP) Interview Questions in Java
Java is an object-oriented programming language, which means that it is designed to work with objects and classes.
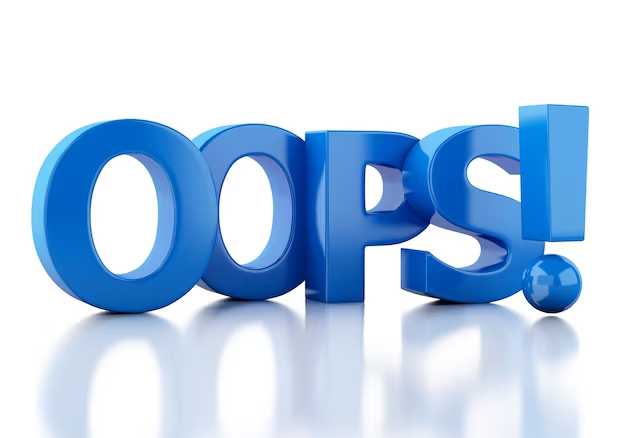
Therefore, it is important to be familiar with OOP concepts and their use in Java. Here are some commonly asked Java OOP interview questions:
1. What is inheritance?
Inheritance is a mechanism that allows a class to inherit properties and methods from another class.
The class that is being inherited from is called the superclass, while the class that is inheriting those properties is called the subclass.
Inheritance allows for code reuse and promotes the creation of more organized and efficient code. So down the line, it makes your life as a programmer a lot easier…
2. What are abstract classes?
An abstract class is a class that cannot be instantiated (basically does nothing by itself). It can only be used as a base class for other classes to inherit from.
Abstract classes are used to define a base set of methods and properties that subclasses must implement. This helps to ensure that all subclasses have a consistent interface and can be used interchangeably.
3. What is polymorphism?
Polymorphism is the ability of an object to take on many forms. In Java, polymorphism is achieved through method overriding and method overloading.
Method overriding is when a subclass provides its own implementation of a method that is already defined in the superclass. Method overloading is when multiple methods with the same name but different parameters are defined in a class.
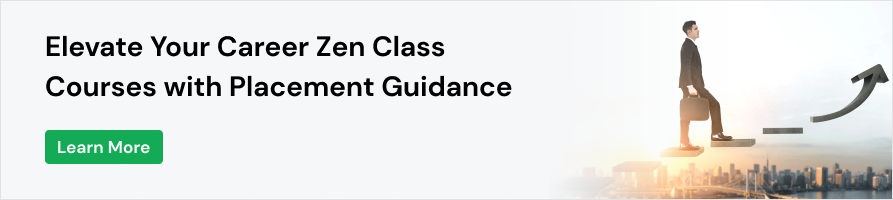
Java Advanced Interview Questions
In addition to OOP concepts, Java has many advanced features and capabilities that employers may ask you about during an interview. Here are some of them:
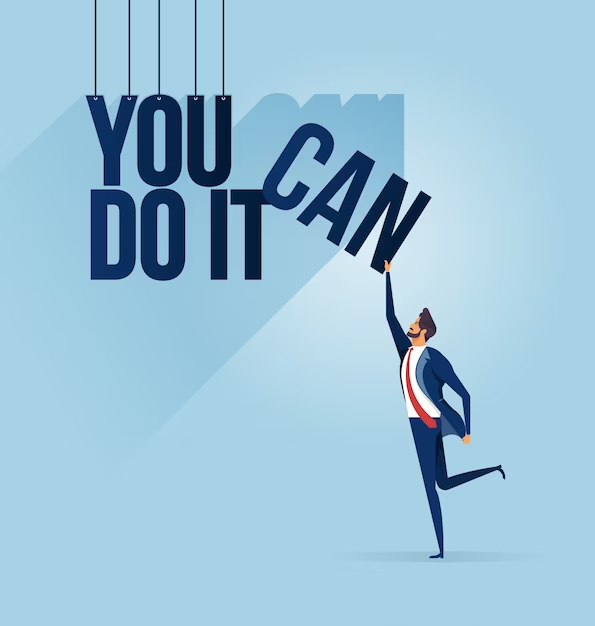
1. What is a Java Virtual Machine (JVM)?
A Java Virtual Machine (JVM) is a piece of software that allows Java programs to run on different platforms without needing to be recompiled.
The JVM provides an environment in which Java programs can execute, and it also manages memory allocation and garbage collection.
2. What is multithreading?
Multithreading is the ability of a program to perform multiple tasks simultaneously. In Java, multithreading is achieved through the use of threads. A thread is a lightweight process that can run concurrently with other threads within the same program.
3. What is serialization?
Serialization is the process of converting an object into a stream of bytes that can be stored in a file or sent over a network. Deserialization is the process of reconstructing the object from the stream of bytes. Serialization is often used in distributed computing and object persistence.
If you wish to be thorough, then, also brush up on these extra questions given below:
- What is the difference between abstract classes and interfaces in Java?
- What is the purpose of the ‘finally’ block in Java?
- What is the difference between ‘==’ and ‘.equals()’ in Java?
Top 5 Must-Know Java Interview Programs for Freshers
Now that we have covered some important Java interview questions, let’s move on to the main topic at hand and one that you must not miss: the top Java programs that you should be familiar with as a fresher.
These programs are designed to test your problem-solving skills and your ability to apply Java concepts to real-world scenarios.
1. Fibonacci Series
In the Fibonacci Series, the next number is said to be the sum of the previous two numbers. Such as 0, 1, 1, 2, 3, 5, 8, 13, 21, etc. The first two numbers of the Fibonacci Series are always 0 and 1.
Here I have illustrated an example without using recursion. This program outputs the first 10 numbers of the Fibonacci Series when executed, you can play with the number by changing the value of the ‘count’ variable and get as many Fibonacci numbers as you desire.
class FibonacciSeries{
public static void main(String args[])
{
int a1=0,b2=1,c3,i,count=10;
System.out.println(a1+" "+b2); //simply printing 0 and 1
for(i=2;i<count;++i) //the loop starts from 2 because 0 and 1 have already been printed
{
c3=a1+b2;
System.out.println(" "+c3);
a1=b2;
b2=c3;
}
}}
2. Check if a Number is Prime
A third commonly asked Java interview program is to check if the given number is a prime. The program takes an integer as input and returns true if the number is prime, and false otherwise. Here is an example solution:
public class PrimeCheck{
public static void main(String args[]){
int a,b=0,flag=0;
int num=3; //this variable represents the number to be checked
b=num/2;
if(num==0||num==1){
System.out.println(num+" is not prime number");
}else{
for(a=2;i<=b;i++){
if(num%a==0){
System.out.println(a+" is not prime number");
flag=1;
break;
}
}
if(flag==0) { System.out.println(a+" is prime number"); }
}
}
}
3. String Palindrome
In this program, we will be checking to see whether a given string is a palindrome or not. A string is said to be a palindrome only if the reverse of that string is exactly the same as the original string. For example, kayak, rotator, etc.
class PalindromePractice{
public static void main(String args[]){
int p,sum=0,tempo;
int num=454; //This variable represents the number to be checked for palindrome
tempo=num;
while(num>0){
p=num%10; //getting the remainder
sum=(sum*10)+p;
num=num/10;
}
if(tempo==sum)
System.out.println("palindrome number ");
else
System.out.println("not palindrome");
}
}
4. Bubble Sort
The bubble sort algorithm is known as the simplest sorting algorithm.
Here, the array is traversed from the first element to the last element step by step. The current element is compared with the next element. If the current element is greater than the next element, only then it is swapped.
public class BubbleSort {
static void bubbleSort(int[] arr) {
int num = arr.length;
int tempo = 0;
for(int i=0; i < num; i++){
for(int j=1; j < (num-i); j++){
if(arr[j-1] > arr[j]){
//swapping elements
tempo = arr[j-1];
arr[j-1] = arr[j];
arr[j] = tempo;
}
}
}
}
public static void main(String[] args) {
int arr[] ={3,60,35,2,45,320,5};
System.out.println("The array before Bubble Sort:");
for(int i=0; i < arr.length; i++){
System.out.println(arr[i] + " ");
}
System.out.println();
bubbleSort(arr); //sorting the array elements using bubble sort method we wrote earlier
System.out.println("The array after Bubble Sort:");
for(int i=0; i < arr.length; i++){
System.out.println(arr[i] + " ");
}
}
}
5. Armstrong Number
In this program, we’re checking to see whether a given number is an Armstrong Number or not.A positive integer is said to be an Armstrong number of the order n if:
abcd… = an + bn + cn + dn + …
So basically a number is an Armstrong number if it is equal to the sum of its digits each raised to the power of the number of digits. For example:
9474 = 94 + 44 +74 +44 = 6561 + 256 + 2401 + 256 =9474. Hence, 9474 is an Armstrong number.
public class ArmstrongCheck {
public static void main(String[] args) {
int num = 1634, ogNum, remainder, final = 0, h = 0;
ogNum = num;
for (;ogNum != 0; ogNum /= 10, ++h);
ogNum = num;
for (;ogNum != 0; ogNum /= 10)
{
remainder = ogNum % 10;
final += Math.pow(remainder, h);
}
if(final == num)
System.out.println(num + " is an Armstrong number.");
else
System.out.println(num + " is not an Armstrong number.");
}
}
Tips for Solving Interview Questions Efficiently
Now that you are familiar with some of the most commonly asked Java interview programs, here are some tips to help you get through the interviews with ease:
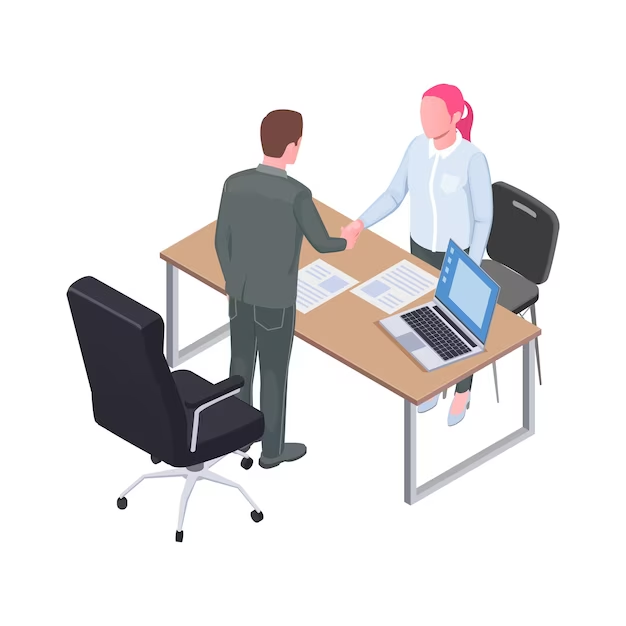
- Practice, practice, practice – the more you practice solving Java programs, the more comfortable and confident you will be during the interview.
- Understand the logic behind the programs – it is important to not just memorize the solutions to the programming questions but to also understand the logic behind them.
- Keep it simple – while it is important to write efficient and optimized code, it is also important to keep it simple and easy to read.
- Show your work – be sure to explain your thought process and the steps you took to arrive at your solution.
- Test your code thoroughly – a must for all programmers: shows how serious you are about your code so get into a habit of testing it regularly.
Additional Resources for Interview Preparation
In addition to the tips provided above, there are many resources available online to help you prepare for Java interviews. Here are some of the most popular ones:
HackerRank – offers coding challenges and contests, as well as interview preparation materials for Java and other programming languages.
GUVI – provides industry-favorite tutorials, practice problems, and access to award-winning practice platforms so that you can master coding in Java in no time. Do refer to this comprehensive Java course.
Common Mistakes to Avoid During Interviews
Finally, here are some common mistakes that you must avoid during these technical interviews:
- Not being familiar with basic Java concepts and syntax: Re-visit all your basics before the interview.
- Not understanding the problem statement or requirements: Read carefully and understand what the problem demands.
- Writing inefficient or overly complex code: Go with the simplest and quickest executing code snippet instead of an exaggerated code.
- Not testing your code thoroughly: Always test your code before submitting or showcasing.
- Not being able to explain your thought process and solution: Be ready to explain how and why this code is your solution.
FAQs
What are the basic programs asked in interviews for freshers in Java?
Some of the most commonly asked programs asked in interviews for freshers in Java are 1) The Fibonacci Series, 2) The Prime Number Check, 3) String Palindrome, 4) Bubble Sort, 5) Merge Sort, 6)Armstrong Number, and 7) Factorial along with a few others.
How to prepare for Java interview for freshers?
You must thoroughly brush up on the following: 1) Java fundamentals, 2) Java OOP Concepts, 3) Multithreading, concurrency, and thread basics, 4) Array, 5) Garbage Collection, and 6) Data Structures and Algorithms.
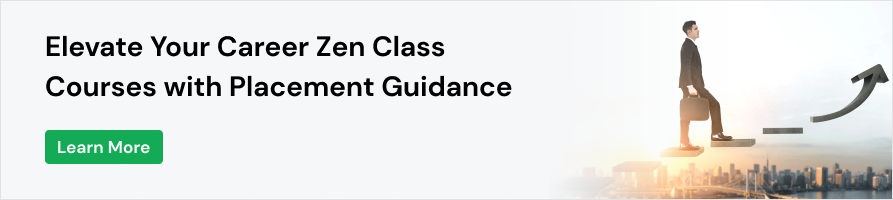
How to practice Java for interview?
You must go through all the basics of Java programming, practice various programs, implement your customized changes and compare the results with other students. Referring to courses by GUVI and practicing on their award-winning platforms will surely help you ace your Java interviews.
Did you enjoy this article?