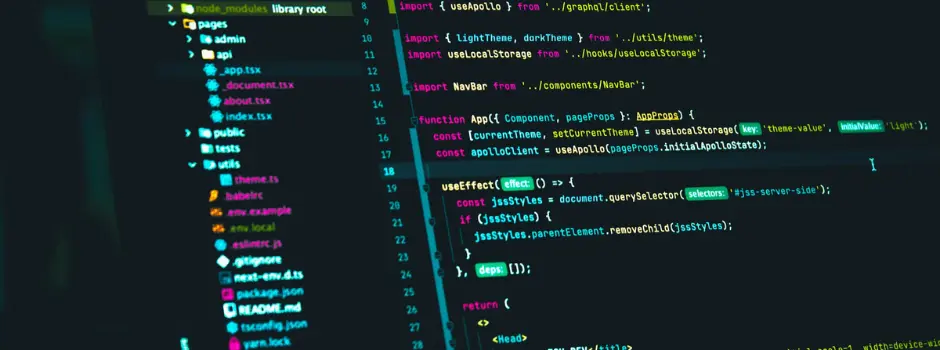
Currency Converter App Using ReactJS: A Step-by-Step Guide
May 09, 2024 4 Min Read 414 Views
(Last Updated)
In today’s global economy, the need for quick and reliable currency conversion is essential for travelers and businesses alike. Building a currency converter app with ReactJS offers a practical way to enhance your skills in UI design, API integration, and state management.
This blog will take you step-by-step through creating a functional app, covering everything from project setup to deployment. Let’s start building!
Table of contents
- What is ReactJS?
- Steps to Create a Currency Converter App Using ReactJS
- Project Structure
- Javascript
- CSS
- Step to Run the Application
- Output
- Conclusion
- FAQs
- How do I fetch and update exchange rates in a React currency converter app?
- What components are essential for a currency converter app in ReactJS?
- How do I handle user input and form validation in a currency converter app?
What is ReactJS?
ReactJS, commonly referred to as React, is an open-source JavaScript library used for building user interfaces, primarily single-page applications. It was developed by Facebook and is maintained by a community of developers. Here are some key features:
- Component-Based Architecture: React’s UI is built using reusable, self-contained components. Each component manages its own state and can be reused across different parts of the application.
- Virtual DOM: React uses a virtual representation of the DOM (Document Object Model) to optimize updates. Changes to the UI are first made to the virtual DOM, which then calculates the most efficient way to update the real DOM, improving performance.
- Declarative Syntax: React allows developers to describe the UI using JSX (JavaScript XML), which is a syntax extension that combines JavaScript and HTML-like code. This makes it easier to design and understand the UI structure.
- Unidirectional Data Flow: React promotes a one-way data flow through the application, making it easier to manage the state and understand how data changes affect the application.
React is popular for building dynamic and responsive user interfaces and is widely used in web development due to its flexibility, performance, and robust ecosystem.
Ready to master ReactJS and build your currency converter app? Enroll now in GUVI’s ReactJS Course and take your web development skills to the next level!
Having explored the foundational concepts of ReactJS, let’s learn how to create a Currency Converter App using ReactJS.
Steps to Create a Currency Converter App Using ReactJS
Let’s learn how to make a Currency Converter App using ReactJS:
You can also learn to use ReactJS to Fetch and Display Data from API in just 5 Simple Steps
Step 1: Set up your React application by executing the following command in your terminal:
npx create-react-app currency-converter
Also Read: Build a Search Component in React [just in 3 simple steps]
Step 2: Navigate to the newly created project folder with this command:
cd currency-converter
Also Read: How to Render an Array of Objects in React? [in 3 easy steps]
Step 3: Install the necessary npm packages for your project by running these commands:
npm install axios
npm install react-dropdown
npm install react-icons
These steps will get your React application ready and equip it with the essential tools to start building the currency converter app.
Also Read: Top ReactJS Interview Questions and Answers Of 2024! [Part-1]
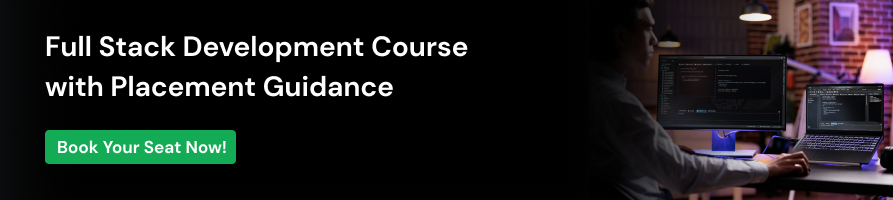
Project Structure
The dependencies section in your package.json will now look like this:
“dependencies”: {
“@testing-library/jest-dom”: “^5.17.0”,
“@testing-library/react”: “^13.4.0”,
“@testing-library/user-event”: “^13.5.0”,
“axios”: “^1.4.0”,
“react”: “^18.2.0”,
“react-dom”: “^18.2.0”,
“react-dropdown”: “^1.11.0”,
“react-icons”: “^4.10.1”,
“react-scripts”: “5.0.1”,
“web-vitals”: “^2.1.4”
}
Example: Add the following code to the specified files:
- App.js: This main component handles all the app’s logic. It utilizes the open-source, no-authentication-required API, ‘currency-api‘, to retrieve a list of available currencies.
- App.css: This file includes the project’s styling.
Also Read: useState() Hook in React for Beginners | React Hooks 2024
Javascript
// App.js
import { useEffect, useState } from 'react';
import Axios from 'axios';
import Dropdown from 'react-dropdown';
import { HiSwitchHorizontal } from 'react-icons/hi';
import 'react-dropdown/style.css';
import './App.css';
function App() {
// Initializing all the state variables
const [info, setInfo] = useState([]);
const [input, setInput] = useState(0);
const [from, setFrom] = useState("usd");
const [to, setTo] = useState("inr");
const [options, setOptions] = useState([]);
const [output, setOutput] = useState(0);
// Calling the api whenever the dependency changes
useEffect(() => {
Axios.get(
`https://cdn.jsdelivr.net/gh/fawazahmed0/currency-api@1/latest/currencies/${from}.json`)
.then((res) => {
setInfo(res.data[from]);
})
}, [from]);
// Calling the convert function whenever
// a user switches the currency
useEffect(() => {
setOptions(Object.keys(info));
convert();
}, [info])
// Function to convert the currency
function convert() {
var rate = info[to];
setOutput(input * rate);
}
// Function to switch between two currency
function flip() {
var temp = from;
setFrom(to);
setTo(temp);
}
return (
<div className="App">
<div className="heading">
<h1>Currency converter</h1>
</div>
<div className="container">
<div className="left">
<h3>Amount</h3>
<input type="text"
placeholder="Enter the amount"
onChange={(e) => setInput(e.target.value)} />
</div>
<div className="middle">
<h3>From</h3>
<Dropdown options={options}
onChange={(e) => { setFrom(e.value) }}
value={from} placeholder="From" />
</div>
<div className="switch">
<HiSwitchHorizontal size="30px"
onClick={() => { flip() }} />
</div>
<div className="right">
<h3>To</h3>
<Dropdown options={options}
onChange={(e) => { setTo(e.value) }}
value={to} placeholder="To" />
</div>
</div>
<div className="result">
<button onClick={() => { convert() }}>Convert</button>
<h2>Converted Amount:</h2>
<p>{input + " " + from + " = " + output.toFixed(2) + " " + to}</p>
</div>
</div>
);
}
export default App;
Ever dreamt of mastering Full Stack Development? Ready to turn that dream into reality? Join GUVI’s Full Stack Development course now and start your journey towards becoming a skilled developer! Enroll now!
Also, Explore 7 Best Reasons to Learn JavaScript | 1 Bonus Point
CSS
/* App.css */
@import url('https://fonts.googleapis.com/css2?family=Pacifico&display=swap');
.App {
height: 100vh;
width: 100%;
display: flex;
align-items: center;
flex-direction: column;
background-image: linear-gradient(120deg, #fdfbfb 0%, #ebedee 100%);
}
.heading {
font-family: 'Pacifico', cursive;
font-size: 35px;
}
.container {
height: auto;
width: 800px;
display: flex;
justify-content: space-around;
align-items: center;
}
input {
padding-left: 5px;
font-size: 20px;
height: 36px;
}
.middle,
.right {
width: 120px;
}
.switch {
padding: 5px;
background-color: rgb(226, 252, 184);
border-radius: 50%;
cursor: pointer;
}
.result {
box-sizing: border-box;
width: 800px;
padding-left: 30px;
}
button {
width: 100px;
height: 30px;
font-weight: bold;
font-size: 20px;
margin-top: 15px;
border: 2px solid forestgreen;
background-color: rgb(226, 252, 184);
cursor: pointer;
}
p,
h3,
button,
.switch {
color: forestgreen;
}
p {
font-size: 30px;
}
Must Know More With Complete CSS Tutorial: Essential Guide to Understand CSS [2024]
Step to Run the Application
To start the application, navigate to the project’s root directory and execute the following command:
npm start
Output
Open your browser and navigate to http://localhost:3000/ to see the app running with the following output:
Conclusion
While this app offers a solid foundation, there’s ample room for enhancements, such as adding support for more currencies, improving the UI, and handling errors gracefully. To continue learning and refining your skills, consult the official React documentation and engage with the developer community, which will help you evolve your projects into sophisticated, feature-rich applications.
Also Read: 10 Best React Project Ideas for Developers [with Source Code]
FAQs
How do I fetch and update exchange rates in a React currency converter app?
To fetch and update exchange rates, you can use an external API like ExchangeRate-API or Open Exchange Rates. Installing an HTTP client like Axios helps with making API calls by allowing you to use GET requests to fetch the latest exchange rate data.
This data can then be stored in the app’s state and updated periodically or whenever a user triggers an update, ensuring that the app always displays the most current rates.
What components are essential for a currency converter app in ReactJS?
An effective currency converter app requires a few essential components. CurrencyInput is necessary for handling user input related to currency selection and entering amounts. ConversionResult displays the calculated conversion results. App.js serves as the main component, managing state, coordinating data flow, and handling API calls.
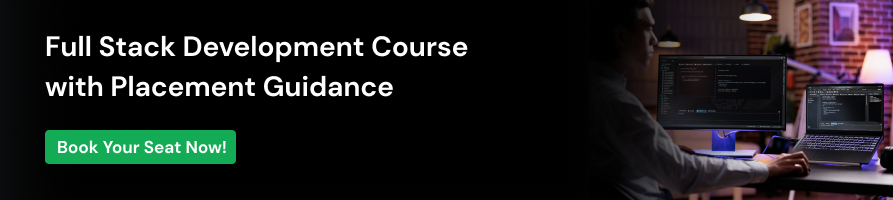
How do I handle user input and form validation in a currency converter app?
React’s controlled components are useful for handling user input. By binding form elements to the app’s state and using event handlers to update the state as users enter input, the app can smoothly process user interactions.
Implementing form validation is important to ensure that the app only accepts valid numerical input, preventing errors like negative values or non-numeric characters from causing issues.
Did you enjoy this article?